Lumibot: Backtesting and Algorithmic Trading Library#
An Easy to Use and Powerful Backtesting and Trading Library for Crypto, Stocks, Options, Futures and FOREX
Looking for a library that makes it easy for you to backtest your trading strategies and easily create algorithmic trading robots? Well you've found us!
Lumibot is a full featured, super fast library that will allow you to easily create trading robots that are profitable in many different asset classes, including Stocks, Options, Futures, FOREX, and more. It is in active development and is constantly being updated to include new features and bug fixes.
If you want to make a fortune with trading, then you need a library that will make it easy for you to check your trading strategies against historical data to make sure they are profitable before you invest in them. Lumibot makes it easy for you to do this (backtest) your trading strategies and easily convert them to algorithmic trading robots.
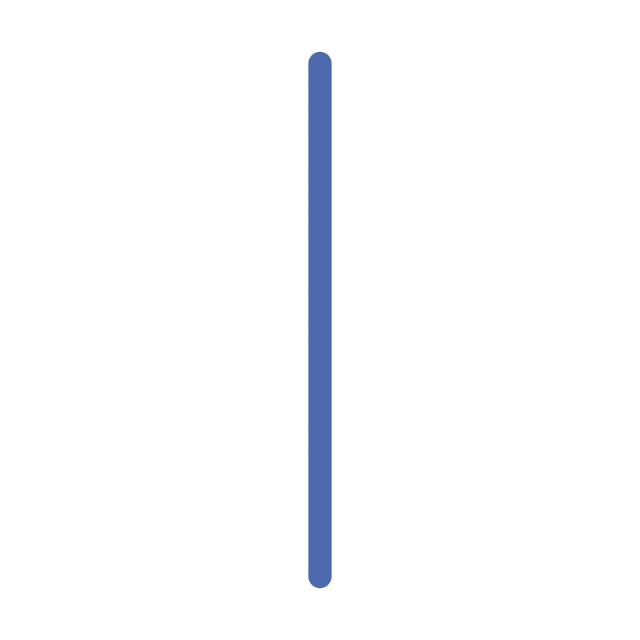
Features
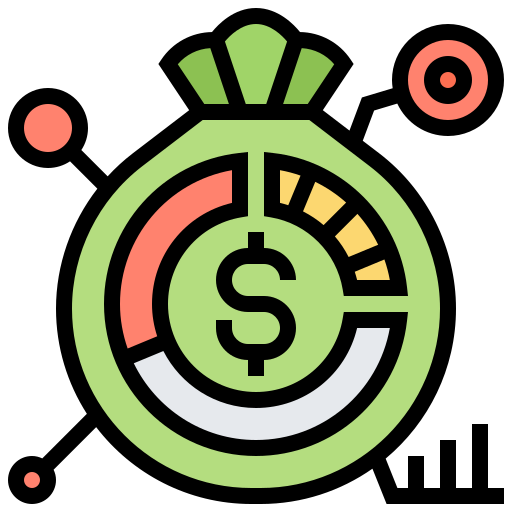
Works with Crypto, Options, Futures, Stocks, and FOREX!
We're one of the few libraries that supports Algorithmic Options trading and backtesting, as well as many other asset classes.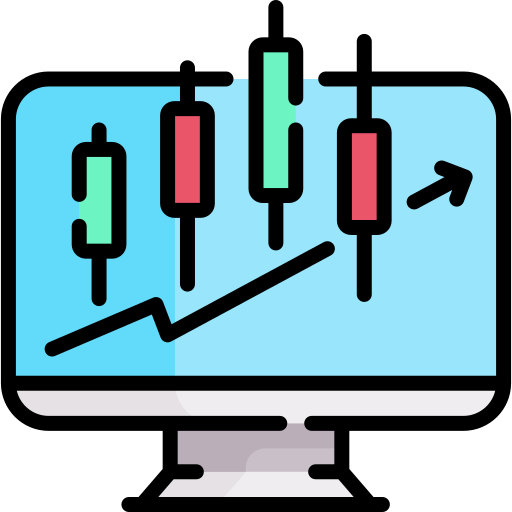
Built in Live Trading
It's very easy to switch between live trading and backtesting, just a few lines of code to go from backtesting to live trading.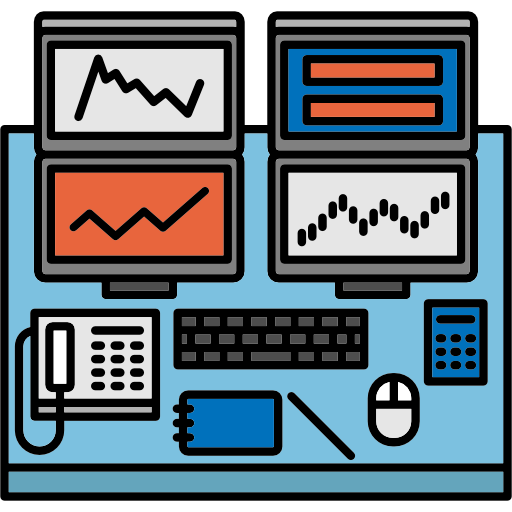
Support For Top Brokers
We support many of the top brokers in the industry, including: Binance, Coinbase, Kucoin, Alpaca, Interactive Brokers and TradeStation. We will also support more brokers in the future.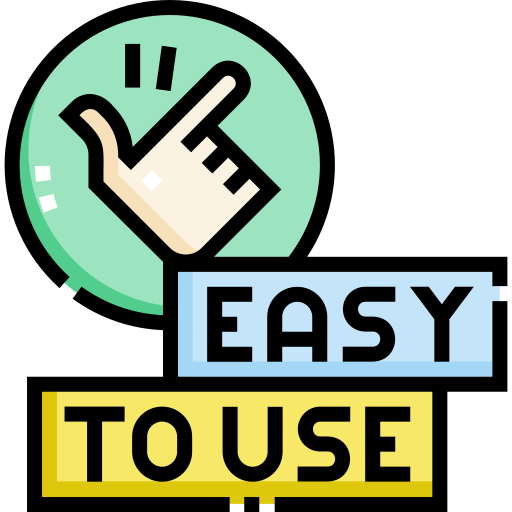
Easy to Use and Get Started
We've built the library to be easy to use and get started in minutes. We also offer courses and tutorials to help you with building your own trading bot. (see our Algorithmic Trading course here)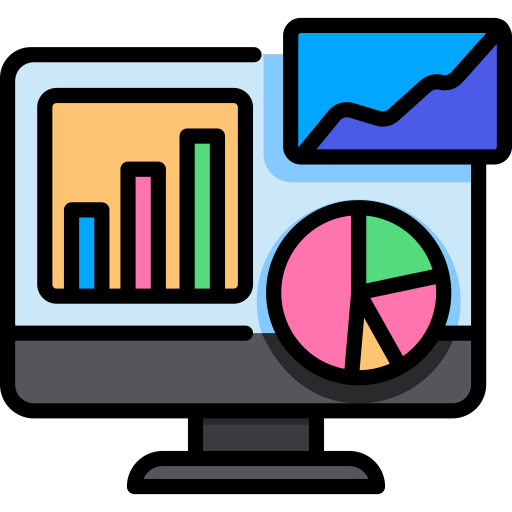
Advanced Analyics Out of the Box
We've built a suite of advanced analytics tools that can be used to analyze and optimize your trading strategies, including interactive charts and more.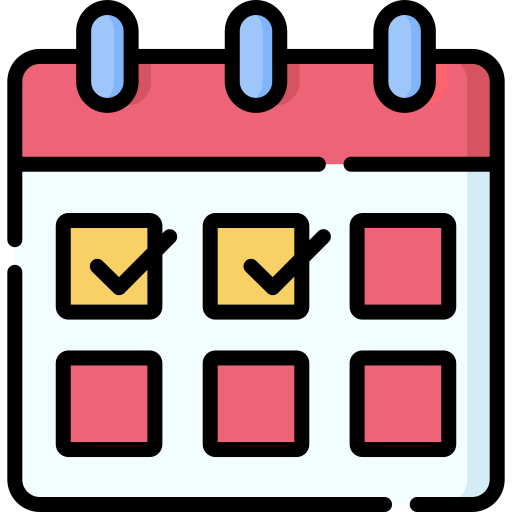
Event Based Backtesting
Our backtesting engine is event-based so that you can see your strategies run as if they were live trading. You don't have to deal with complicated and confusing vector math to see your strategies run.Getting Started
Getting Started#
After you have installed Lumibot on your computer, you can create a strategy and backtest it using free data available from Yahoo Finance, or use your own data. Here’s how to get started:
Step 1: Install Lumibot#
Note
Ensure you have installed the latest version of Lumibot. Upgrade using the following command:
pip install lumibot --upgrade
Install the package on your computer:
pip install lumibot
Step 2: Create a Strategy for Backtesting#
Here’s some code to get you started:
from datetime import datetime
from lumibot.backtesting import YahooDataBacktesting
from lumibot.strategies import Strategy
# A simple strategy that buys AAPL on the first day and holds it
class MyStrategy(Strategy):
def on_trading_iteration(self):
if self.first_iteration:
aapl_price = self.get_last_price("AAPL")
quantity = self.portfolio_value // aapl_price
order = self.create_order("AAPL", quantity, "buy")
self.submit_order(order)
# Pick the dates that you want to start and end your backtest
backtesting_start = datetime(2020, 11, 1)
backtesting_end = datetime(2020, 12, 31)
# Run the backtest
MyStrategy.backtest(
YahooDataBacktesting,
backtesting_start,
backtesting_end,
)
Step 3: Take Your Bot Live#
Once you have backtested your strategy and found it to be profitable on historical data, you can take your bot live. Notice how the strategy code is exactly the same. Here’s an example using Alpaca (you can create a free Paper Trading account here in minutes: https://alpaca.markets/).
from lumibot.brokers import Alpaca
from lumibot.strategies.strategy import Strategy
from lumibot.traders import Trader
ALPACA_CONFIG = {
"API_KEY": "YOUR_ALPACA_API_KEY",
"API_SECRET": "YOUR_ALPACA_SECRET",
"PAPER": True # Set to True for paper trading, False for live trading
}
# A simple strategy that buys AAPL on the first day and holds it
class MyStrategy(Strategy):
def on_trading_iteration(self):
if self.first_iteration:
aapl_price = self.get_last_price("AAPL")
quantity = self.portfolio_value // aapl_price
order = self.create_order("AAPL", quantity, "buy")
self.submit_order(order)
trader = Trader()
broker = Alpaca(ALPACA_CONFIG)
strategy = MyStrategy(broker=broker)
# Run the strategy live
trader.add_strategy(strategy)
trader.run_all()
Important
Remember to start with a paper trading account to ensure everything works as expected before moving to live trading.
All Together#
Here’s the complete code:
from datetime import datetime
from lumibot.backtesting import YahooDataBacktesting
from lumibot.brokers import Alpaca
from lumibot.strategies import Strategy
from lumibot.traders import Trader
ALPACA_CONFIG = {
"API_KEY": "YOUR_ALPACA_API_KEY",
"API_SECRET": "YOUR_ALPACA_SECRET",
"PAPER": True
}
class MyStrategy(Strategy):
def on_trading_iteration(self):
if self.first_iteration:
aapl_price = self.get_last_price("AAPL")
quantity = self.portfolio_value // aapl_price
order = self.create_order("AAPL", quantity, "buy")
self.submit_order(order)
trader = Trader()
broker = Alpaca(ALPACA_CONFIG)
strategy = MyStrategy(broker=broker)
# Run the strategy live
trader.add_strategy(strategy)
trader.run_all()
Or you can download the file here: https://github.com/Lumiwealth/lumibot/blob/dev/lumibot/example_strategies/simple_start_single_file.py
Additional Resources#
If you would like to learn how to modify your strategies, we suggest that you first learn about Lifecycle Methods, then Strategy Methods, and Strategy Properties. You can find the documentation for these in the menu, with the main pages describing what they are, then the sub-pages describing each method and property individually.
We also have some more sample code that you can check out here: https://github.com/Lumiwealth/lumibot/tree/dev/lumibot/example_strategies
We wish you good luck with your trading strategies. Don’t forget us when you’re swimming in cash!
Need Extra Help?#
If you need extra help building your strategies and making them profitable, Lumiwealth has you covered. By visiting Lumiwealth, you not only learn how to use the Lumibot library but also gain access to a wealth of highly profitable algorithms.
Our strategies have shown exceptional results, with some achieving over 100% annual returns and others reaching up to 1,000% in backtesting. Join us to learn from our expertise and take your trading to the next level.
Why Choose Lumiwealth?
By joining Lumiwealth, you'll learn to:
- ✔️ Create and implement your own trading algorithms
- ✔️ Access a library of high-performing strategies
- ✔️ Learn from experts with proven track records
- ✔️ Achieve potentially high returns on your investments
Important
Build Trading Bots with AI
Want to create trading bots without writing code? Visit BotSpot - our platform for building, testing, and deploying trading strategies using AI!
Create strategies using natural language
Backtest on historical data
Deploy to live markets
Join a community of algorithmic traders
Get started at https://botspot.trade/
Table of Contents#
- Home
- Build Bots with AI
- GitHub
- Discord Community
- Get Pre-Built Profitable Strategies
- Deployment Guide
- Secrets Configuration
- Broker Configuration
- Tradier Configuration
- Tradovate Configuration
- Alpaca Configuration
- Coinbase Configuration
- Kraken Configuration
- Kucoin Configuration
- Binance Configuration
- Bitmex Configuration
- Bybit Configuration
- OKX Configuration
- Interactive Brokers Configuration
- Interactive Brokers-Legacy Configuration
- Schwab Configuration
- Bitunix Configuration
- DataBento Configuration
- ProjectX Configuration
- General Environment Variables
- What is Lumibot?
- Lumiwealth
- Getting Started With Lumibot
- Step 1: Install the Package
- Step 2: Import the Following Modules
- Step 3: Create an Alpaca Paper Trading Account
- Step 4: Configure Your API Keys
- Step 5: Create a Strategy Class
- Step 6: Instantiate the Trader, Alpaca, and Strategy Classes
- Step 7: Backtest the Strategy (Optional)
- Step 8: Run the Strategy
- Adding Trading Fees
- Profiling to Improve Performance
- Lifecycle Methods
- Summary
- def initialize
- def on_trading_iteration
- def before_market_opens
- def before_starting_trading
- def before_market_closes
- def after_market_closes
- def on_abrupt_closing
- def on_bot_crash
- def trace_stats
- def on_new_order
- def on_partially_filled_order
- def on_filled_order
- def on_canceled_order
- def on_parameters_updated
- Variable Backup & Restore
- Strategy Methods
- Strategy Properties
- Entities
- Futures Trading
- Backtesting
- Brokers
- Alpaca
- Bitunix
- Crypto Brokers (Using CCXT)
- Interactive Brokers
- Interactive Brokers Legacy
- ProjectX
- Schwab
- Prerequisites
- Environment variables
- First-time login
- Token life-cycle
- Supported functionality
- Example
.env
- Example strategy snippet
- Troubleshooting
- API Credentials
- Token Life-cycle & Auto-refresh
- Creating an App & Getting Keys
- OAuth2 Authentication Flow
- Sandbox vs Production
- Supported Assets & Order Types
- Market Data
- Rate Limits & Token Expiry
- Known Issues & Best Practices
- Example Strategy
- Support & Contact
- Tradier
- Tradovate